Django And Django Rest Framework: Beginners' Guide
Django And Django Rest Framework: Beginners' Guide
Welcome to the world of Django and Django Rest Framework! If you're excited to learn the ins and outs of these powerful tools, you're in the right place.
In this beginners' guide, we'll take you on a journey through the fundamentals of Django and Django Rest Framework, breaking down complex concepts into easy-to-understand pieces.
What is Django and Django Rest Framework?
Django is a high-level Python web framework that follows the model-view-controller (MVC) architectural pattern. It provides a fast and efficient way to build web applications by emphasizing reusability, maintainability, and scalability. Django handles many common web development tasks, such as URL routing, database management, and form handling, allowing developers to focus on building the application's core features.
The Django Rest Framework (DRF) is an extension to Django that allows developers to easily build RESTful APIs. It provides a set of powerful tools and abstractions, including serializers, viewsets, and authentication mechanisms, that help developers write clean and efficient code for exposing their Django models as RESTful endpoints. DRF simplifies common tasks such as handling HTTP requests and responses, pagination, filtering, and authentication, making it an ideal choice for building web services and APIs with Django.
To learn more about Django and Django Rest Framework, continue reading the comprehensive guide below.
Django and Django Rest Framework: Beginner's Guide
Welcome to the beginner's guide to Django and Django Rest Framework! In this comprehensive article, we will explore the basics of Django, a high-level Python web framework, and its extension, Django Rest Framework. Whether you are new to web development or have experience with other frameworks, this guide will provide you with the knowledge and skills to get started with Django and Django Rest Framework. Let's dive in!
Introduction to Django
Django is a powerful and popular Python web framework that follows the Model-View-Template (MVT) architectural pattern. It provides developers with a set of tools and conventions to build robust and scalable web applications quickly. Django's batteries-included approach means that it comes with many built-in features and libraries, reducing the need for developers to reinvent the wheel.
Some of the key features of Django include:
- Object-Relational Mapping (ORM) for easy database interactions
- Automatic admin interface generation
- Template engine for flexible and dynamic web page rendering
- User authentication and authorization
- URL routing system
- Forms handling
Benefits of Django
Django offers several benefits for beginners and experienced developers alike:
1. Efficiency and Productivity
With Django's built-in features and conventions, developers can focus on the application logic rather than spending time on repetitive tasks. The framework's admin interface generation, ORM, and template engine enable rapid development and code reuse.
2. Security
Django incorporates security best practices, such as protection against common web vulnerabilities like cross-site scripting (XSS), cross-site request forgery (CSRF), and SQL injection attacks. It also provides built-in user authentication and authorization mechanisms.
3. Scalability
Django follows a scalable architecture, allowing developers to easily handle high traffic and large-scale applications. It includes features like database connection pooling, caching, and asynchronous task processing through integrations with tools like Redis and Celery.
Django vs. Other Web Frameworks
When choosing a web framework, it's essential to consider your project requirements and the strengths of different frameworks. Here's how Django stacks up against other popular frameworks:
1. Django vs. Flask
Flask is another popular Python web framework, but it follows a minimalist "micro" approach. While Flask offers flexibility and simplicity, Django provides a more comprehensive solution with built-in features like an ORM, admin interface, and authentication system. Django may be a better choice for larger and complex projects, while Flask is suitable for smaller, lightweight applications.
2. Django vs. Ruby on Rails
Ruby on Rails (RoR) is a web framework similar to Django but written in Ruby. Both frameworks follow the MVC architectural pattern and offer robust solutions for web development. The choice between Django and RoR often depends on the programming language preference and the existing expertise of the development team.
Getting Started with Django
Now that you understand the basics of Django, let's get started with setting up your development environment and creating your first Django project:
1. Install Python and Django
To use Django, you need to have Python installed on your system. Visit the Python website and download the latest version of Python. Once Python is installed, you can install Django using pip, the Python package manager. Run the following command in your terminal:
pip install django
2. Creating a Django Project
Once Django is installed, you can create a new Django project by running the following command:
django-admin startproject myproject
This will create a new directory called "myproject" with the necessary files and folders for a Django project.
3. Running the Development Server
To start the development server and see your Django project in action, navigate into the project directory and run the following command:
python manage.py runserver
You should see output indicating that the development server is running. Open your web browser and visit http://localhost:8000/
to see your Django project's default landing page.
Introduction to Django Rest Framework
Django Rest Framework (DRF) is an extension of Django that simplifies the process of building Web APIs. It provides a set of powerful tools and serializers to easily create, test, and consume RESTful APIs. DRF integrates seamlessly with Django, making it an excellent choice for developing API endpoints for new or existing Django projects.
Benefits of Django Rest Framework
Let's explore the advantages of using Django Rest Framework for building Web APIs:
1. Serialization
DRF offers powerful serialization capabilities, allowing you to convert complex Python objects into JSON, XML, or other formats. Serializers handle object validation, input deserialization, and output serialization, making it easy to work with data transmitted over the network.
2. Authentication and Permissions
DRF provides a range of authentication classes and permission types to secure your API endpoints. You can choose from session-based authentication, token-based authentication, or even implement your custom authentication methods. Additionally, permissions can be set to limit access to resources based on user roles or other criteria.
3. Viewsets and Routers
DRF introduces the concept of viewsets, which combine the functionality of views and URL routing. Viewsets allow you to define common CRUD (Create, Retrieve, Update, Delete) operations for a model or a set of related models. DRF also provides routers to automatically generate the required URL patterns for your viewsets.
Integration with Django
Integrating DRF into your Django project is straightforward. Here's how you can get started:
1. Install Django Rest Framework
Install Django Rest Framework using pip:
pip install djangorestframework
2. Add DRF to Django's Installed Apps
In your Django project's settings file, locate the INSTALLED_APPS
list and add 'rest_framework' to the list. This ensures that DRF is included in your project.
3. Define Serializers for Your Models
Create serializers that define the structure and behavior of your API endpoints. Serializers handle the conversion between Python objects and JSON representations.
4. Define Viewsets and Routers
Create viewsets to handle various actions and define router URLs to automatically map the URLs to the appropriate viewset methods.
Best Practices for Django and Django Rest Framework
As you gain experience with Django and Django Rest Framework, keeping a few best practices in mind can help you write cleaner and more maintainable code:
1. Use Class-Based Views
Django encourages the use of class-based views (CBVs) over function-based views (FBVs) for increased code reusability and readability. CBVs allow you to define common behavior in parent classes and inherit from them to create more specific views.
2. Write Unit Tests
Writing tests is crucial to ensure the stability and correctness of your Django projects. Use the built-in testing framework and write unit tests for your models, views, and API endpoints to automate the testing process and catch bugs early.
3. Follow the RESTful Principles
When building Web APIs with Django Rest Framework, try to adhere to RESTful design principles. Use the appropriate HTTP methods (GET, POST, PUT, DELETE) for different operations, apply meaningful URL patterns, and structure your responses to provide the expected data to clients.
4. Implement Pagination and Filtering
If your API endpoints return large amounts of data, implement pagination to improve performance and prevent overwhelming clients with excessive data. Additionally, consider implementing filtering and search capabilities to allow clients to retrieve specific subsets of data efficiently.
5. Secure your APIs
Ensure that your APIs are secure by implementing appropriate authentication and authorization mechanisms. Use HTTPS for secure communication, and consider rate limiting and throttling to prevent abuse and unauthorized access.
Statistic
Django and Django Rest Framework have become incredibly popular among developers. As of 2021, Django is the fourth most loved web framework according to the Stack Overflow Developer Survey, with a significant community and a wide range of resources available. Additionally, Django Rest Framework has over 16,000 stars on GitHub, highlighting its popularity and widespread adoption in the industry.
Now that you have a solid foundation of Django and Django Rest Framework, it's time to start building your own web applications and APIs. Remember to explore the official documentation and join the vibrant communities around these frameworks to enhance your skills and knowledge. Happy coding!
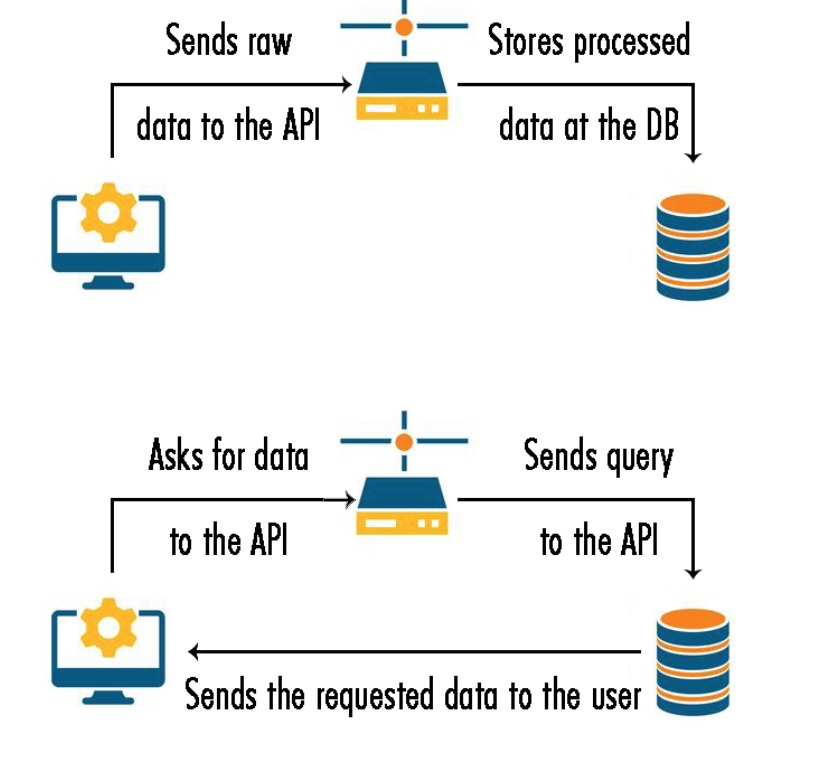
Frequently Asked Questions
Welcome to our Frequently Asked Questions section about Django and Django Rest Framework for beginners. Below, we have compiled some common questions to help you get started with these frameworks.
1. What is Django and why should I use it for web development?
Django is a high-level Python web framework that allows developers to quickly build efficient and secure web applications. It follows the Model-View-Controller (MVC) architectural pattern and provides built-in tools and libraries for tasks such as database management, URL routing, and user authentication. Using Django can significantly speed up the development process and ensure code quality and maintainability.
Whether you are a beginner or an experienced developer, Django's simplicity, extensive documentation, and strong community support make it an excellent choice for web development projects.
2. What is Django Rest Framework and why is it useful?
Django Rest Framework (DRF) is a powerful toolkit for building Web APIs using Django. It extends Django's functionality to handle HTTP requests and responses, making it easier to develop RESTful APIs. DRF provides features such as serialization, authentication, automatic URL routing, and support for various response formats like JSON and XML.
Using DRF, developers can create robust APIs that can be consumed by other applications, allowing data to be easily exchanged between systems. It simplifies the process of building scalable and maintainable web services, making it an essential tool for modern web development.
3. How do I install Django and Django Rest Framework?
To install Django, you can use pip, the package installer for Python. Open your command line interface and run the following command:
pip install django
For installing Django Rest Framework, you can also use pip. Use the following command:
pip install djangorestframework
Make sure you have Python and pip installed before running these commands.
4. How can I create a basic Django project?
Once Django is installed, you can create a new project by running the following command in your command line interface:
django-admin startproject projectname
This will create a new Django project with the given name. You can navigate into the project folder and start building your web application by defining models, views, and URL mappings.
5. How can I create a basic Django Rest Framework API?
To create a basic API using Django Rest Framework, you need to follow these steps:
1. Define your models in Django, representing the data you want to expose through the API.
2. Create serializers, which translate your models into formats like JSON.
3. Define views, which handle the API requests, perform actions on the models, and return serialized data.
4. Map the views to URLs using Django's URL routing mechanism.
By following these steps, you can create a fully functional API using Django Rest Framework, enabling your application to communicate with other systems or clients.
Comments
Post a Comment